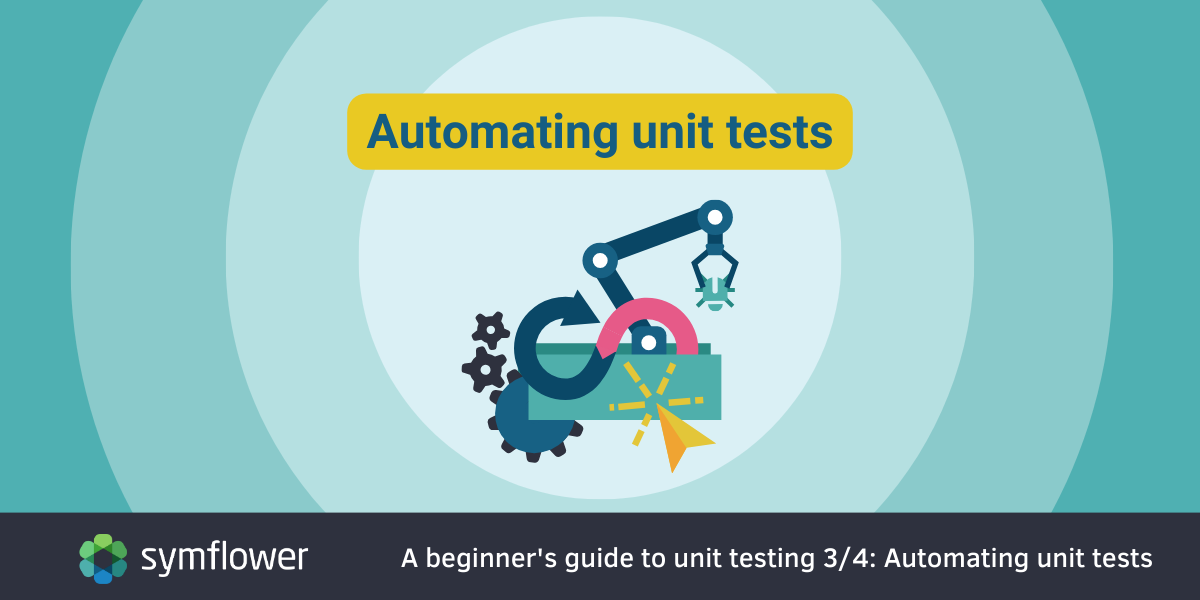
Part 3 of our introduction to unit testing tackles the topic of automating unit tests in your application development workflow.
In our series about unit testing, we’ve already covered what unit tests are and how to use them. This post will go into more detail about using automation to streamline the creation and execution of unit tests. In the next (last) post of this series, we’ll dive deep into some best practices for unit testing.
Check out all the other parts of this series:
- A beginner’s guide to unit testing 1/4: What is unit testing?
- A beginner’s guide to unit testing 2/4: How to use unit tests?
- A beginner’s guide to unit testing 4/4: Unit testing best practices
Unit tests in your software development workflow
As you know by now, unit tests are small tests used to check the smallest components of code (e.g. a function, method, or line of code). Since they are so small and targeted, you’ll be using tons of unit tests. That’s why automation is especially important for unit testing to save time and effort.
Most teams make unit test execution a part of their CI/CD pipeline to make sure their tests are run and that regressions are caught with each new code change. But automation can also help you with the creation of unit tests so that it doesn’t take as much manual effort. Let’s zoom in on both of those topics.
Why automate unit test execution?
Unit tests contribute to higher software quality, enabling the early discovery of problems. And automation reduces the time and effort costs of doing so.
By making unit test execution a part of your automated CI workflow, you’ll get feedback on code quality earlier in the process of development when problems are faster, cheaper, and easier to fix. Automating unit testing through an established testing framework and process helps boost efficiency and collaboration in your team, leading to enhanced productivity. Building unit testing into your CI/CD pipeline also helps prevent regressions.
In the following sections, we’ll cover some practical aspects of automating unit tests in Java only.
Automating the creation of unit tests in Java
There are widely used tools to automate test execution (see the next section), but you would traditionally still write unit tests manually.
For the first time ever, you can now automate the writing of unit tests. Some use AI (code completion) tools like GitHub Copilot or even ChatGPT to create unit tests. While not a bad approach per se, this creates some extra manual work for you as a developer.
A basic tool stack for automating Java unit test execution
Once you have your unit tests written, it’s time to automate their execution. There are various tools out there to support this.
🦾 The top Java unit testing frameworks & tools
Looking to unit test Java code? Here are the most popular unit testing frameworks and some useful tools to support your unit testing efforts!
JUnit
JUnit is the most fundamental unit testing framework used by the majority of Java developers. It is open-source and provides a structure for creating automated tests, executing them, as well as verifying and reporting on their results.
👯♀️Wondering which unit testing framework to use?
Check out our comparison of JUnit vs TestNG!
We’ve published two comprehensive post series on writing JUnit test cases and a few of the most important JUnit testing tips and tricks. Make sure you check out these resources if you’re using JUnit! If you choose to use Symflower, you’ll notice that it automatically generates JUnit tests to make things still easier for you.
Mockito
To be able to test isolated units of your application, you’ll need to mock some interactions. Mockito is the most popular mocking framework in the Java world.
It lets you create a range of test doubles including fakes, stubs, mocks, and spies as needed. Symflower uses Mockito for mocking in its unit tests. We provide an example of using Mockito in one of our earlier blog posts, so again, if you’re using the most popular frameworks, Symflower saves you a great deal of time and effort.
Build tools: Maven or Gradle
You will obviously need a build tool like Maven or Gradle for your application. Both can simplify unit test execution by letting you quickly and consistently execute unit tests, and then report on their results.
Maven’s Surefire plugin was designed to run unit tests. And we wrote a whole tutorial on running JUnit 5 tests with Gradle, so whichever build tool you’re using, you’re covered.
Selenium
Selenium is also open-source and is mainly used to test web applications. It supports browser automation and you can use it to automate not just unit tests but also integration and functional testing. You can use Selenium in combination with either JUnit or TestNG, making it a sound choice regardless of your testing/development environment.
Tools for CI/CD pipeline automation
You’ll want to run unit tests for each development branch. It’s also important to make sure that branches can only be merged in case all unit tests are passed. The best way to do that is to automate unit test execution in your Continuous Integration / Continuous Delivery workflow.
Tools like Gitlab CI/CD and JetBrains' TeamCity or Atlassian’s BitBucket can help automate processes in your CI/CD pipeline to make sure (unit) testing forms an integral part of your workflow. Practical know-how on how to do this is outside the scope of this post, but be sure to let us know if that’s a topic you’re interested in!
Summary
Unit testing is a core development practice that all developers should use. With smart automation, it’s not even as resource-intensive as it used to be, so there’s really no point in ignoring the importance of unit testing.