A beginner's guide to unit testing 4/4: Unit testing best practices
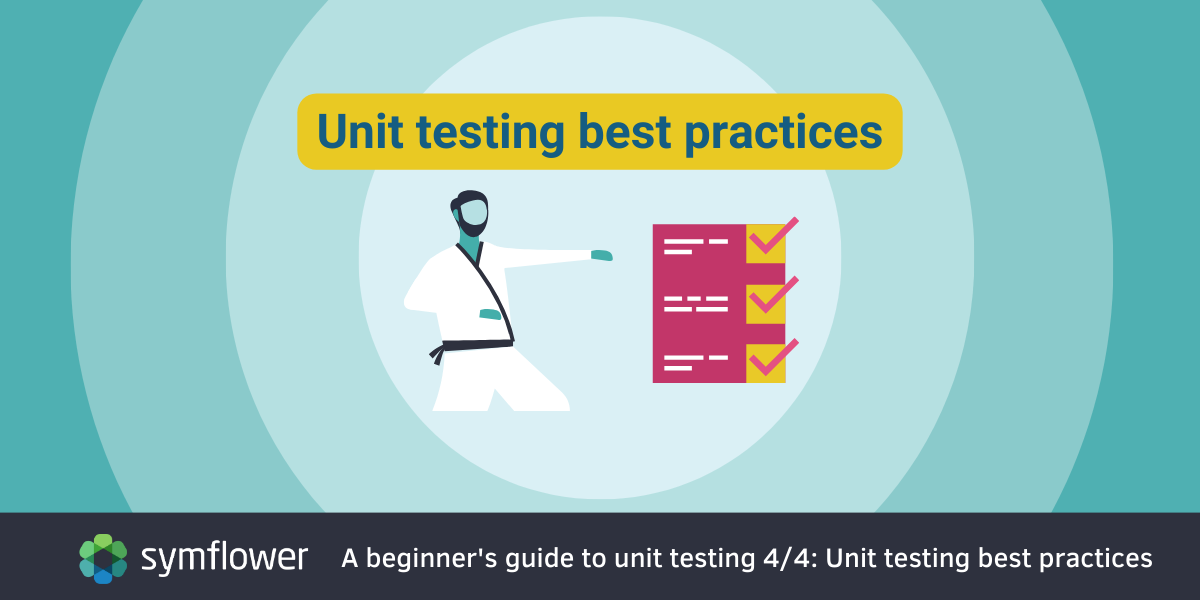
In part 4 of our guide to unit testing, we list a few of the most important best practices for writing effective unit tests.
Unit tests are a powerful tool to enhance the robustness of your code. Having a good unit test suite in place generally vastly improves productivity and reduces bug fixing times. But that’s only really true if they are done right. Fail to follow some of the established best practices for unit testing and you may find that unit tests can be a serious productivity drainer not just for you, but anyone working with your code. Unnecessarily testing things that don’t need to be tested, failing to remove dependencies and then spending time debugging, or creating a test suite without a clear structure will lead to wasted time. We rounded up a few key tips to help you do unit testing right.
Check out all the other parts of this series:
- A beginner’s guide to unit testing 1/4: What is unit testing?
- A beginner’s guide to unit testing 2/4: How to use unit tests?
- A beginner’s guide to unit testing 3/4: Automating unit tests
Unit testing best practices
In the following sections, we’ll detail a few vital things to keep in mind as you start writing unit tests.
π€ New to unit testing?
Check out our introductory guide to writing JUnit test cases and flex those JUnit skills with our advanced techniques for writing JUnit test cases!
Isolate your unit tests
Unit tests should be run in a controlled test environment and are expected to provide deterministic results. Make sure your unit tests focus on a single piece of code and can be successfully run independently of external resources (such as databases). Use mocks and stubs to remove any external dependencies for your tests.
π€ What are the best mocking frameworks for Java?
Check out our comparison of Mockito vs EasyMock vs JMockit!
You’ll also want to ensure that your unit tests don’t influence each other e.g. the results of one test should not affect another test.
Ensure readability
With the consistent use of unit tests, documentation is less of a burden since the tests aptly describe what the code is supposed to do. Ensure readability in your testing code to help other team members better understand the tested functionality.
It’s a great idea to use the tried-and-tested Arrange-Act-Assert pattern outlined in part 2. Here’s a quick reminder:
- Arrange: Set up the necessary objects (inputs and expected outputs)
- Act: Call the method being tested
- Assert: Check actual vs expected results
Aim for simplicity in your unit tests and refrain from unnecessary nesting. Try to provide useful error messages to reduce debugging time for yourself and other team members working with your code.
Organize your tests logically
Use a sound naming convention for your unit tests to help others understand your test suite in no time (and of course to make things easier to navigate for your future self). Add descriptive names for your methods, test cases, and test files and organize them based on a logical structure.
A general best practice for organizing tests is to follow this naming convention:
MethodName_StateUnderTest_ExpectedBehavior
Rather than just going with the “Testβ suffix that most IDEs add to your test methods, try to come up with a name that conveys what the test is verifying.
πͺ Best practices for naming and storing test files for Java and Go
Curious about proven ways to name and store test files for Java and Go unit tests? Check out our post!
Focused assertions
Keep in mind that a unit test should focus on a single business case. If you have more than one assertion in a unit test, consider splitting it into two tests. Keep it short, simple, easy to read, and quick to run.
Test data initialization
Where to write your initialization code can cause some headaches. The convention is to:
- Place all general initialization code (e.g. initialization code that is common to all your tests) in the
@Before
or@BeforeClass
methods.@Before
is run before each test method, and@BeforeClass
before each class. - Initialization code that is relevant only for specific test cases should be placed in the test case.
Automate unit testing in your CI
Passing unit tests should be a requirement for any branch before they are merged, let alone deployed to production. In fact, at Symflower, we don’t even start reviewing an MR unless all unit tests are passed. If there are failing unit tests, that means there’s still work to be done.
Overall, it’s a wise idea to make unit testing part of your integration pipeline to make sure no broken code is shipped. In general, make use of automation throughout the process of managing unit tests.
π Why write unit tests when you can generate them?
Symflower automates unit test template creation to ease the burden of unit testing. Its beta feature can even generate complete test suites together with test values that explore all paths in your code! Try it in your IDE.
Summary: best practices for effective unit testing
Keeping to these best practices should help you make unit testing an efficient and effective part of your workflow. That concludes our introductory series to unit testing. Missed the previous parts of this series? Check them out to hit the ground running with unit testing, and send us your thoughts if you think the series can be improved in any way!
- A beginner’s guide to unit testing 1/4: What is unit testing?
- A beginner’s guide to unit testing 2/4: How to use unit tests?
- A beginner’s guide to unit testing 3/4: Automating unit tests
Make sure you never miss any of our upcoming content by signing up for our newsletter and by following us on Twitter, LinkedIn or Facebook!