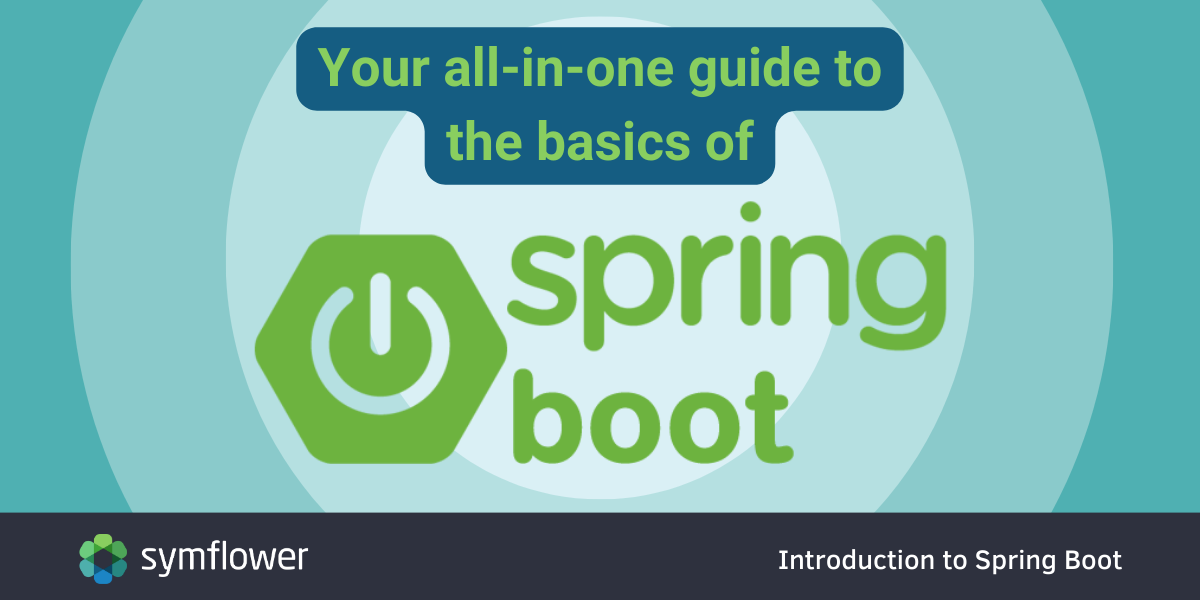
Heard all the good things about Spring Boot but not quite sure what it’s all about? It’s OK, we’ve got you covered: this post is your fundamental guide to Spring Boot!
Introduction: what is Spring Boot?
Java Spring Boot is an extension to the Spring framework that makes the powerful features of this framework easier to access. Spring Boot takes things one step further than Spring’s dependency injection feature, as it can automatically take care of configuration for a variety of elements.
ℹ️ What is dependency injection?
“It is a process whereby objects define their dependencies (that is, the other objects they work with) only through constructor arguments, arguments to a factory method, or properties that are set on the object instance after it is constructed or returned from a factory method. The container then injects those dependencies when it creates the bean."
Source: Spring Framework documentation
A useful feature of Spring Boot is that it offers opinionated ‘starter’ dependencies that help simplify the build configuration so that you can quickly create production-grade Spring applications (see more on starter dependencies below).
Overall, Spring Boot simplifies the creation of standalone applications using the Spring framework by automatically creating boilerplate configurations for setting up the application
👨💻 Clarifying the Spring Framework
Wondering about the differences between Spring vs Spring Boot vs Spring Web MVC vs Spring WebFlux? Check out our post:
Understanding the Spring framework: Spring vs Spring Boot vs Spring Web MVC vs Spring WebFlux
What are the main features of Spring Boot?
Spring Boot provides a flexible means to configure Java beans, XML configurations, and database transactions.
As mentioned above, Spring Boot provides valuable help in three key areas:
- Autoconfiguration: both the underlying Spring framework and any 3rd party packages you’re using will be initialized with pre-set dependencies, saving you the time and effort of manual configuration.
- An opinionated approach to configuration: Spring Boot will automatically take care of adding and configuring starter dependencies. The framework saves you time by automatically identifying the right packages to install and the default values to use.
- The ability to create standalone applications: Using Spring Boot lets you build applications that don’t rely on an external web server (applications that you can “just run”, in Spring’s own terminology). The application is easily launched on any platform.
🤖 Automate test creation
Looking to write unit and integration tests for your Spring Boot application? Check out Symflower, our plugin that generates all the boilerplate you’ll need for testing Spring components! Try Symflower in your IDE.
Spring Boot tutorial: getting started with Spring Boot
So how does Spring Boot work? Think of it as a project initializer: if you’re about to develop Spring applications like REST backends, microservices for a website, or even entire mobile applications, using Spring Boot makes your job easier.
To bootstrap a Spring Boot application in a very short time, you can use a tool called Spring Boot Initializr. It’s an easy-to-use web form that you can fill in with basic information about your project. The Spring Boot Initializr will generate a web application automatically configured based on your settings.
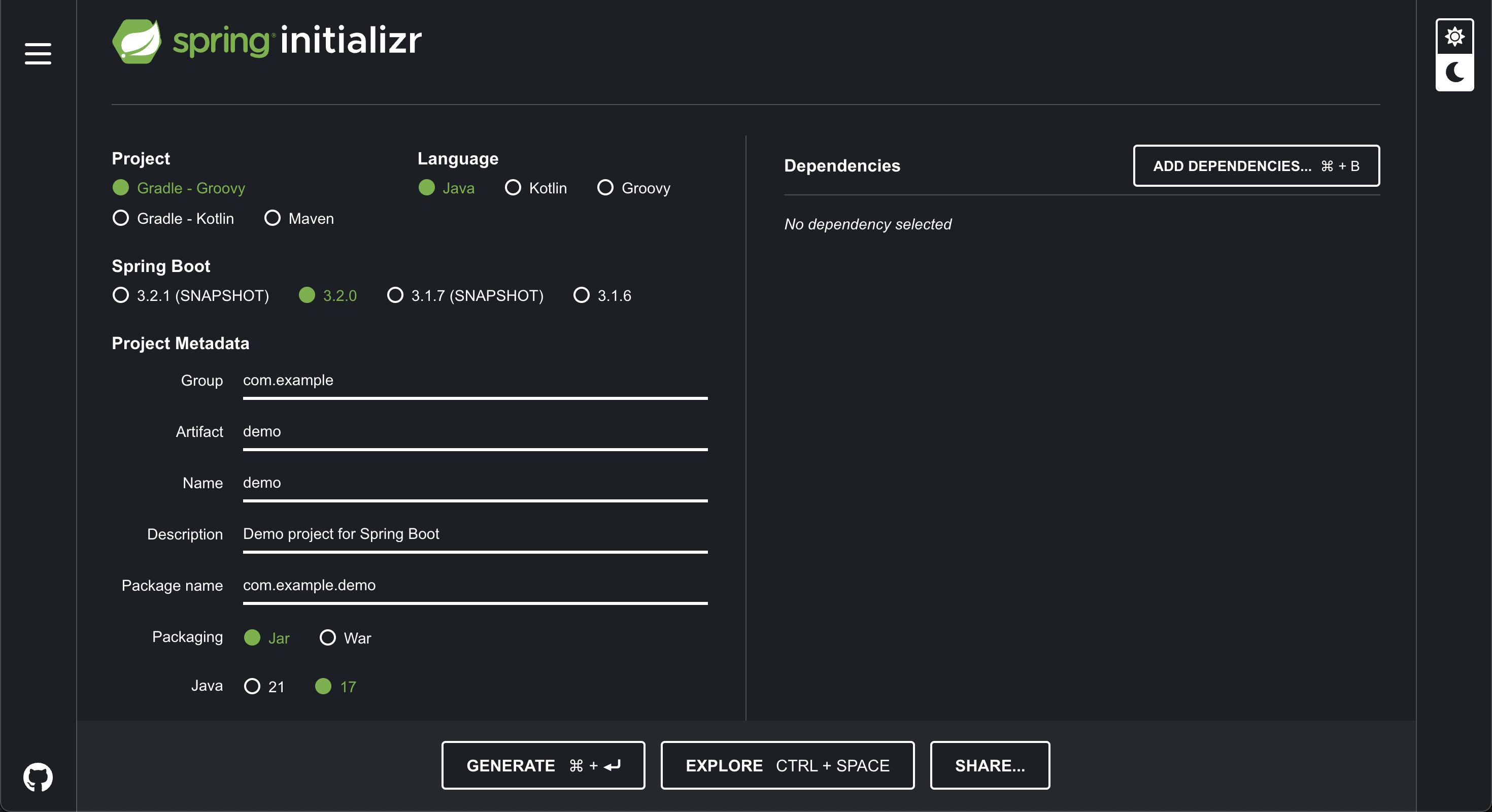
- Using the
@SpringBootApplication
annotation to create an application class makes sense because it combines three key annotations:@EnableAutoConfiguration
,@ComponentScan
, and@SpringBootConfiguration
. Let’s see what each of these do: @EnableAutoConfiguration
: This one adds dependencies by enabling Spring Boot’s auto-configuration capabilities.@ComponentScan
: It enables Spring Boot to scan the package where the application is located for autoconfiguration.@SpringBootConfiguration
: This one just indicates that the class annotated with it provides application configuration. As opposed to the simple@Configuration
, this annotation supports automatic configuration detection, which is useful for unit and integration tests.
So a smart way to get started with Spring Boot is to just add @SpringBootApplication
to your application class (the class that has your application’s main method) to get access to all of that Spring Boot goodness outlined above.
To further simplify build configuration, Spring Boot offers a variety of starter dependencies (called Spring Boot Starters) that cover a range of typical use cases. These starter dependencies let you easily add jars to your classpath so you won’t have to manually enter (or copy-paste) tons of dependency descriptions. Some examples of Spring Boot Starters include:
Spring Boot Starter name | Description |
---|---|
Spring-boot-starter-webflux | Starter for building WebFlux applications using Spring Framework’s Reactive Web support. |
Spring-boot-starter-web | Starter for building web, including RESTful, applications using Spring MVC. Uses Tomcat as the default embedded container. |
Spring-boot-starter-logging | Starter for logging using Logback. Default logging starter. |
Spring-boot-starter-test | Starter for testing Spring Boot applications with libraries including JUnit Jupiter, Hamcrest and Mockito. |
To use a starter, you’ll just include the relevant dependency in your project. For instance, if you wanted to use Spring and JPA to access a database, you’d include the spring-boot-starter-data-jpa dependency in your project as follows:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
See the full list of starter dependencies in Spring Boot’s documentation. You can actually even configure your own custom starters – see how that’s done in Spring Boot’s documentation.
After all that is done, Spring Boot will analyze your classpath and all the beans you’ve configured. If it detects any missing elements, those will be added automatically.
🔍 Testing in Spring Boot
Once you have a Spring Boot application, you’ll need to test it too. Wondering how? Check out our guide:
Best practices for testing Spring Boot applications: a complete guide
Pros and cons of Spring Boot
Spring Boot is popular for good reason. It has a range of benefits for developers looking for a fast way to get started with building (web) applications. Despite all its advantages, there are a few downsides, too.
Advantages of Spring Boot:
- The main benefit of Spring Boot is that it provides a simple and fast way to build applications without the need to configure standard dependencies.
- It also helps reduce code length and configuration time.
- It provides easy access to the features of the Spring framework without the lengthy manual configuration normally required.
- It is easy to launch, manage, and customize, and doesn’t require XML configuration.
- Spring Boot is great if you’re developing cloud applications in a DevOps environment.
- It also supports Tomcat, Jetty, and Undertow application servers out of the box, meaning faster and more efficient deployment.
- What’s more, it provides production-ready features including a range of metrics, health checks, and externalized configuration.
On the other hand, there are a few disadvantages, too.
Disadvantages of Spring Boot:
- Convenience comes at the expense of control. Because of autoconfiguration, using Spring Boot can result in unused dependencies being created. That can be a problem if you’re working on a large-scale project like a complex enterprise application because it can result in unnecessarily large deployment files.
- The general consensus is that Spring Boot is not great when building big monolithic applications. It’s awesome for quickly building microservices and other small applications.
- It’s not easy to convert a legacy project to Spring Boot. If you’re starting from scratch, you may want to consider using Spring Boot, but if you’re working on an existing project, it may not be worth it to switch.
So that’s it about the basics of Spring Boot! At this point, you should have a sound understanding of how Spring Boot works and whether it’s right for your project. If you’re curious about some examples on how all this works out in practice, look no more:
- The Spring documentation offers a getting started guide with code examples.
- Another good resource is IBM’s tutorial that helps you hit the ground running with starters, opinions, and an executable JAR file structure in Spring Boot.
Make sure you never miss any of our upcoming content! Sign up for our newsletter and follow us on Twitter, LinkedIn or Facebook!