Those new to the Spring ecosystem can easily get confused about the differences between Spring, Spring Boot, Spring Web MVC, and Spring WebFlux. In this post, we clear it all up!
A guide to the Spring framework
In the development of software, application frameworks are used to provide infrastructure support for code libraries to make core programming easier. Application frameworks support code reusability and include tools that help developers build and combine the various components of software applications.
In the Java world, open-source Spring is the most popular application framework, with 60% of developers using it for the production of their applications. (According to Snyk’s JVM Ecosystem Report 2021, Spring Boot is used by over 57% of Java developers, while over 28% of Java developers are using Spring MVC.)
However, the Spring ecosystem consists of several modules and add-ons, and the term “Spring” is often used to refer to the whole family of Spring projects. So we set out to clarify the differences between Spring and Spring Boot, and we’ll also cover two other often-used Spring terms that can cause confusion: Spring Web MVC and Spring WebFlux!
What is the Spring framework?
The Spring Framework is the foundation of all other Spring projects such as the Spring Web MVC framework, the Spring WebFlux reactive web framework, the Spring Boot extension for auto-configuration and creating microservices. This illustration shows the relationship between elements of the Spring ecosystem:
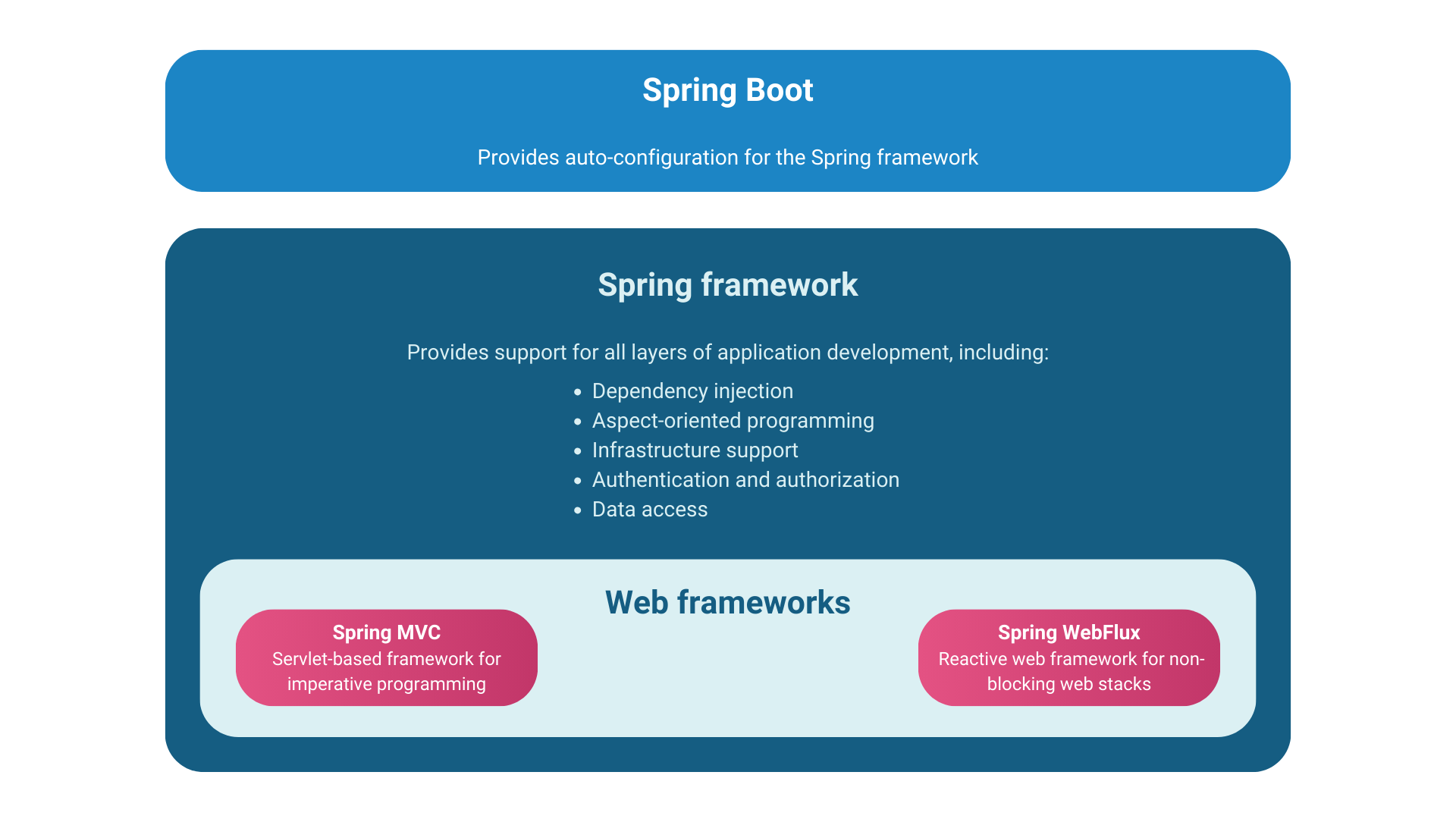
Started in 2003, Spring is a lightweight Java development framework used to help adopt and apply Java in an enterprise environment. It provides basic support for different application architectures. The framework covers messaging, transactional data and persistence, and web. It also includes two web frameworks: Spring MVC and Spring WebFlux.
Basically, in Spring’s own words, the framework “provides a comprehensive programming and configuration model for modern Java-based enterprise applications”, regardless of the eventual deployment environment. A key element of Spring is infrastructural support. Think of it as the provider of “plumbing” for enterprise applications so that developers can focus on the more value-added work of figuring out business logic.
What are the key features of Spring?
One of the core features that make Spring so useful is dependency injection (a means to apply the Inversion of Control principle).
Inversion of control (IoC) is a software design pattern where the custom-coded parts of the application take the flow of control from a generic framework (e.g. rather than the custom code calling reusable libraries to manage generic tasks, IoC has the framework call the custom code). Dependency injection basically lets developers provide objects with their dependencies for easy decoupling. This makes it easier to test such classes as it enables the use of stubbing and mocking in unit tests.
One key term around all things Spring-related is the concept of “beans”. A bean is an object in your application that is instantiated, assembled, and managed by Spring’s IoC container. Through dependency injection, Spring’s container will take care of injecting all the required dependencies of your bean when creating it. A unique feature of Spring is that it lets developers decouple dependency configuration and specification from the application’s logic.
Spring is very useful for building serverless applications, asynchronous applications, and scalable microservices with security being a key focus. The framework contains modules and features that facilitate application development. Basically, Spring contains all the building blocks you need for modern web applications, including:
- Managing authentication & authorization
- Providing a framework for aspect-oriented programming
- Embracing the MVC principle
- Managing transactions
- Managing data access to relational databases and mappers (Spring also supports NoSQL databases)
- Providing support for unit and integration testing
Learn more about testing in Spring boot in one of our earlier blog posts:
Spring also includes two types of web frameworks: the Servlet-based Spring Web MVC framework and the Spring WebFlux reactive web framework. Learn more about web frameworks in an upcoming section!
The benefits of using Spring
So why is Spring so popular? The answer lies in the principles guiding the design of Spring: while being lightweight, it is very flexible, offers intuitive APIs, and provides backward compatibility for easier maintenance. The framework supports all layers of application development, enables loose coupling through dependency injection, and allows for easy testing.
Further benefits of Spring include:
- Supporting declarative programming e.g. expressing computation logic without describing the control flow.
- Providing flexibility to configure Spring via both XML and annotation configurations, depending on your choice.
- Providing middleware services through Spring IoC or Aspect-Oriented Programming (AOP) for instance, when developing distributed applications.
Spring Web Framework comparison
As mentioned before, Spring offers two web frameworks: Spring Web MVC and Spring WebFlux.
- Spring Web MVC is the web framework that was originally included in the Spring framework. It was purpose-built for the Servlet API and Servlet containers.
- Spring WebFlux was added later as a reactive-stack web framework.
Web MVC and WebFlux can coexist and work as optional modules, so you can use none, either one, or both as your application requires.
🤔 Spring Web MVC vs Spring WebFlux
Read our comprehensive overview and comparison of WebFlux and Spring MVC.
What is Spring Web MVC?
Spring Web MVC is an often-used web framework within the Spring framework. MVC is an abbreviation for Model-View-Controller, the 3 key components used to design web applications:
- Model: Sets the rules and logic, and includes the data structures of the application.
- View: Defines the UI logic and produces an HTML output that the user will see in their web browser.
- Controller: This component provides access to the application behavior that you would normally define via a service interface. It interprets user input, validates it, and translates it into a model that will be rendered to the user by the View component.
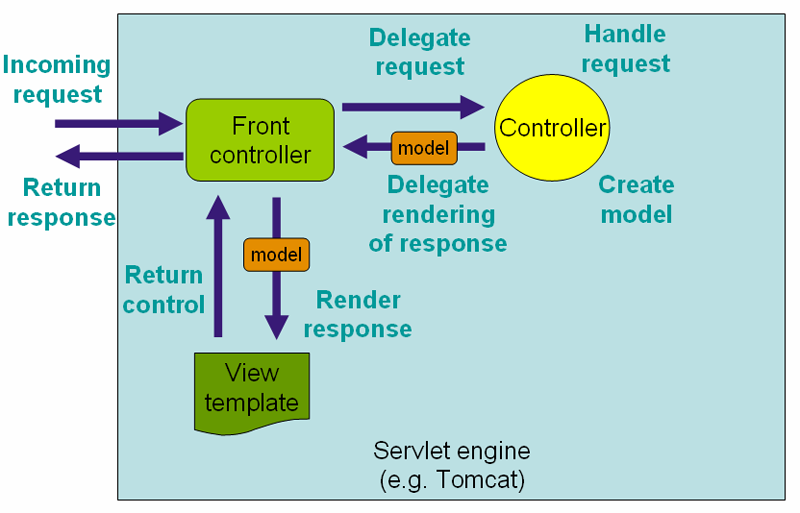
Through these core components, Spring Web MVC allows you to efficiently separate business logic, presentation logic, and navigation logic for your application, which comes in handy when building modular web applications.
Spring Web MVC can be used alone, as part of the Spring framework to build Java applications. However, with Spring Boot, the process will require less manual configuration, making it faster and easier. See more on Spring Boot below.
What is Spring WebFlux?
Spring WebFlux is a reactive and fully non-blocking framework that is able to handle concurrency and enables efficient scaling. Reactivity is important for interoperability in the case of more complex applications that need a high-level, function-rich API to compose asynchronous logic.
WebFlux uses the Reactor library, which has a strong focus on server-side Java, so Reactor is a core dependency. But WebFlux can actually also work with other reactive libraries via Reactive Streams.
It’s great to use WebFlux because it:
- Supports a wide range of servers (including Netty, Tomcat, Jetty, Undertow, and Servlet containers)
- Offers a choice of two programming models (annotated controllers and functional web endpoints)
- And lets you choose a reactive library to use (Reactor, RxJava, or a different one).
What is Spring Boot?
Spring Boot is an extension to the Spring framework that provides automatic configuration of a range of required elements. Think of Spring Boot as an automated version of traditional Spring.
Need an introduction to Spring Boot?
Read our all-in-one guide to the basics of Spring Boot.
Using Spring Boot makes it easy to create standalone, production-grade applications using the Spring framework that, in Spring’s brilliant phrasing, you can “just run”. With Spring Boot, you won’t have to worry about writing boilerplate configurations for setting up the application – all that will be taken care of automatically.
What are the key features of Spring Boot?
Spring Boot provides an opinionated take on Spring, offering ‘starter' dependencies and auto-configuration of both Spring and 3rd party libraries to hit the ground running. This makes Spring Boot a great tool to bootstrap Spring applications from scratch.
Spring Boot is basically a project initializer for Spring applications that facilitates the development of microservices for websites and entire mobile-based applications. After checking your classpath and your configured beans, Spring Boot will try to determine and add missing elements automatically. It provides the default codes and annotation-based configuration automatically to accelerate the development of applications. Production-ready features including a range of metrics, health checks, and externalized configuration make Spring Boot especially useful.
Spring Boot works with popular embedded servlet containers including Tomcat, Jetty, and Undertow, but Spring Boot applications can also be deployed to any container compatible with Servlet 5.0+.
Benefits of using Spring Boot
The primary advantage of Spring Boot is that it offers a simple and very fast way to build and deploy applications. Using it helps reduce the length of your code and provides easy access to the benefits of the Spring Framework.
The auto-configuration offered by Spring Boot saves the time and effort costs of writing code, reducing development time and simplifying configuration. Spring Boot lets you build applications in a way that’s DevOps and cloud-friendly. It is easy to launch, manage, and customize, and doesn’t require XML configuration.
Disadvantages of Spring Boot
Spring Boot is generally not considered suitable for complex, large-scale, monolithic enterprise applications because of all the unused dependencies it creates. These can lead to large deployment files that mean unnecessary clutter.
It can also take quite long to replace a legacy system with Spring Boot applications, and the costs may outweigh the benefits.
Comparison: differences between Spring vs Spring Boot
Spring | Spring Boot | |
---|---|---|
Use case | Mostly used for building enterprise Java applications | Primarily used for building REST APIs |
Main goal | Facilitate enterprise Java development through Java EE (Enterprise Edition) | Reduce code length and make web application development easier and faster |
Core feature | Dependency injection | Autoconfiguration |
Types of application | Loosely coupled applications | Standalone applications |
Server setup | Manual setup | Built-in or embedded servers (Tomcat, Jetty, Undertow) |
Configuration | Manual | Automatic |
In-memory database support | No support | Support for in-memory database like H2 |
XML configuration | Required | Not required |
Plugins for build systems like Maven, Gradle, etc | Not provided | Plugins provided |
- https://rollbar.com/blog/spring-vs-spring-boot-whats-the-difference/
- https://www.interviewbit.com/blog/spring-vs-spring-boot/
Summary: Spring vs Spring Boot?
So should you use plain Spring or Spring Boot? As always, there’s no definite answer for everyone, as it depends on your needs.
Spring Boot provides auto-configuration and “sane” defaults for various Spring components with a focus on building REST APIs. Another advantage of Spring Boot is that you do not need in-depth knowledge of Spring to just get going with a simple application (after all, most configurations are done out of the box).
For most new projects, it makes sense to use Spring Boot and to manually tweak the auto-configurations only where it is necessary. That is especially true for applications intended to be deployed on a cloud or container-based architecture. For building large, enterprise Java applications, Spring might be a better option.
Whether you’re using Spring or Spring Boot to build your application, you’ll definitely want to test it. That’s where a test generator like Symflower will come in handy. This IDE plugin gives you generated test templates in an instant, and can also automatically create entire test suites to test all possible paths in your code. Once a test suite is generated, Symflower helps you discover and reproduce runtime exceptions by giving you test-backed code diagnostics suggestions right in your editor. It’s free to use in your IDE – why not give it a try? Download the Symflower plugin now.
Make sure you never miss any of our upcoming content by signing up for our newsletter and by following us on Twitter, LinkedIn or Facebook!